
How to implement Schema.org with Next.js Project
Search Engines like Google, Bing, Yahoo, etc. has their own algorithms to rank the web pages. They consider many factors like content, keywords, website speed, etc. to rank the web pages. You can optimize your website to rank higher in search engines by following some SEO techniques. they go through the content of the page and try to understand the context of the page. You can help them by providing explicit clues about the meaning of a page, by including schema structured data.
In this tutorial, we will learn how to add a schema to our nextjs project. we'll cover how to add Structured Data using the JSON-LD format. so let's get started.
What is Schema.org structured data?
Schema.org is a collection of vocabulary (or schemas) used to apply structured data markup to web pages and content. Correctly applying schema can improve SEO outcomes through rich snippets. Schema.org is a collaboration between Google, Bing, Yandex, and Yahoo! to help you provide the information their search engines need to understand your content and provide the best search results possible at this time. For example, you can markup your ecommerce product pages with variants schema to help Google understand product variations and display them in search results. Schema.org is a type of microdata that makes it easier for search engines to parse and interpret the information on your web pages more effectively so they can serve relevant results.
JSON-LD Format
JSON-LD(JSON for Linking Data) is a way to create a network of standards based, machine readable data across websites. It is a lightweight data format, easy to read and write.
For example, a news article can be described using below JSON-LD :
1<script type="application/ld+json">
2{
3"@context": "https://schema.org",
4"@type": "NewsArticle",
5"headline": "Title of a News Article",
6"image": [
7"https://example.com/photos/1x1/photo.jpg",
8"https://example.com/photos/4x3/photo.jpg",
9"https://example.com/photos/16x9/photo.jpg"
10],
11"datePublished": "2015-02-05T08:00:00+08:00",
12"dateModified": "2015-02-05T09:20:00+08:00",
13"author":
14[
15{
16"@type": "Person",
17"name": "Jane Doe",
18"url": "https://example.com/profile/janedoe123"
19},
20{
21"@type": "Person",
22"name": "John Doe",
23"url": "https://example.com/profile/johndoe123"
24}
25]
26}
27</script>
The JSON-LD contains standard markups to describe the content of the article. You can find all the markups at schema.org
Add JSON-LD to a Next.js page
Go to the route directory of your page and create a layout.tsx (or .jsx if you prefer) for your page and define the JSON-LD by including it in the layout using the Next.js Script tag.
If you are using typescript, I recommend you use the npm package schema-dts to import the types for each structured data type.
1import Script from "next/script";
2import { Person, WithContext } from "schema-dts";
3const jsonLd: WithContext<Person> = {
4 "@type": "Person",
5 name: "Grace Hopper",
6 disambiguatingDescription: "American computer scientist",
7 birthDate: "1906-12-09",
8 deathDate: "1992-01-01",
9 awards: [
10 "Presidential Medal of Freedom",
11 "National Medal of Technology and Innovation",
12 "IEEE Emanuel R. Piore Award",
13 ],
14};
15export default function PageLayout({ children }: { children: React.ReactNode }) {
16 return (
17 <>
18 {" "}
19 <Script
20 id="person-schema"
21 type="application/ld+json"
22 dangerouslySetInnerHTML={{ __html: JSON.stringify(jsonLd) }}
23 />{" "}
24 {children}{" "}
25 </>
26 );
27}
If your web page contains multiple types/schema, like Articel, FAQ and Product for instance, include a <Script /> tag for each type.
Validate your page
Checking if your page is correctly formatted is important. search engines will not index your page if the structured markup is not correctly added. To verify, you can use any of below tool :
Schema Markup Validator
Google Rich Results Test tool.
Paste the URL of your website, and test. If the markup is correct, you’ll see a page like below.
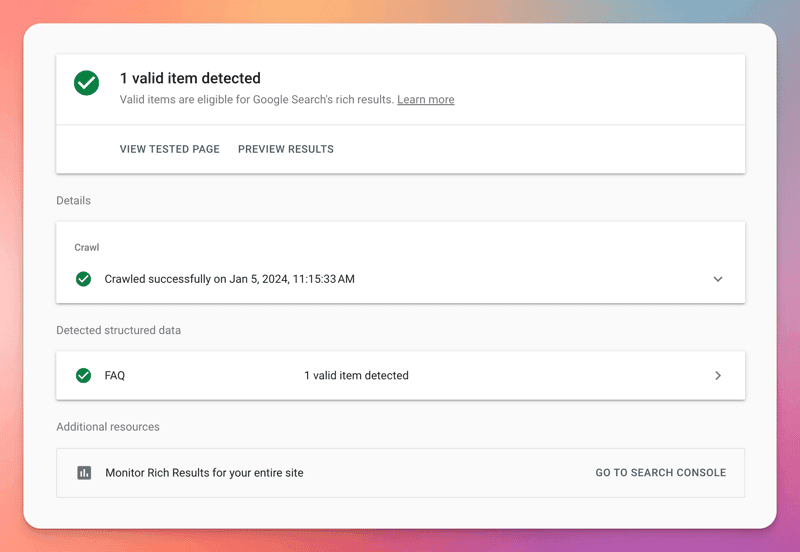
Conclusion
The Schema.org Markup helps search engines understand the content of your article. It is a type of structured data that you can add to your HTML to improve the way your page is represented in search results. This can help your article appear in rich results, which are search results that include additional information like images, ratings, and other details and can make your article stand out in search results.
If you find this tutorial helpful, please share it with your friends and colleagues. Don't forget to follow me :-)